Context handler function
Introduction
The Context handler function is used to control the conversational flow of an action. You can change the order in which the params are prompted to the users in runtime. You can also skip some params, do custom validations of the param values, and also ask for a confirmation before action execution in the runtime.
The Context handler function can override the order in which the static params are defined. If the context handler function is enabled, the bot will prompt the parameters in the order as dictated by the context handler function and not by the order you have declared while defining the params in the Catalyst console.
Invocation Point
Once ConvoKraft detects the action of the user input, the possible param values that are present in the user input will be detected, then the bot will prompt the first missing param to the user. When a user replies to the param prompt, ConvoKraft will invoke the context handler function. The context handler function is invoked for every param that is prompted in the conversation. If an action doesn’t have any params, the context handler function will be executed once before the execution function.
Input Arguments
In addition to the system defined input arguments, the following input arguments are also available for the Context handler function :
Argument | Deluge data type | Description |
---|---|---|
userInput | String | Latest reply from the user |
previousParam | Map | The param that is last prompted to the user. The latest reply of the user is a value to this param. |
Return Value
The Context handler function returns a map in the following format :
copy{ "todo":"prompt", "prompt":{ "param_name":"<name of the param to be prompted>", "prompt_msg":"<the text to be displayed as a prompt message>", "validation_failure_message":"<the text to be displayed if the user submits invalid input>", "options":[ ], "buttons":[ ], "fields":[ ], }, "confirm":{ "message":"Are you sure you want to cancel?" }, "assign":{ "clientData":{ "<key1>":"<value1>", "<key2>":"<value2>" //add the client data as necessary } } }
As depicted above, the following keys can be present in the map returned from the Context handler function :
todo
This key is used to indicate what a Convokraft bot should do after the execution of the context handler function. It can only take one of the following values. Also, please note that this key is mandatory.
- prompt : Instructs the bot to prompt a param to the user.
- confirm : Instructs the bot to ask for a final confirmation for invoking the Execution function of the action.
- execute : Instructs ConvoKraft to invoke the Execution function.
prompt
This key contains a map that specifies what param is to be prompted to the user and is used only if todo is set as prompt.
If this key is not provided even if todo is set as prompt, the bot will prompt the next param as per the order of the params defined in the console. Thus this key can be skipped if we have to use the default order of the params definition. The map value of this key contains the following attributes :
-
param_name : The name of the param to be prompted. The param name must be one of the existing params defined in the console.
-
prompt_msg : This can be used to override the prompt message of the param defined while creating the param in the Catalyst console.
validation_failure_message — The custom message to be displayed if the input given by the user is invalid for the prompted param. This key is optional.
-
options : The list of options of the single and multiple selection list params, from which the user can make a choice.
- id : The unique ID of the option.
- label : The text to be displayed as the option’s label.
- preview : The additional information to be displayed for options in the single and multiple selection list params. Option previews will be available only when the input mode of the single and multiple selection list params is Visual. The preview key is optional.
- header : The header lists the properties of the single and multiple selection list param options. The header object should contain the following attributes in the below format :
copy{ "id":"<the unique id for the option>", "label":"<the text to be displayed as the options label>", "preview":{ "header":{ "title":"<the text to be displayed as the option previews title>", "description":"<the text to be displayed as the option previews description>", "subheading":"<the text to be displayed as the subheading to the options preview>", "image":{ "url":"<the url of the preview image>" } } } }
If the header object is configured with these appropriate attributes for the options, then the preview will be displayed when the user clicks the info icon on each option.
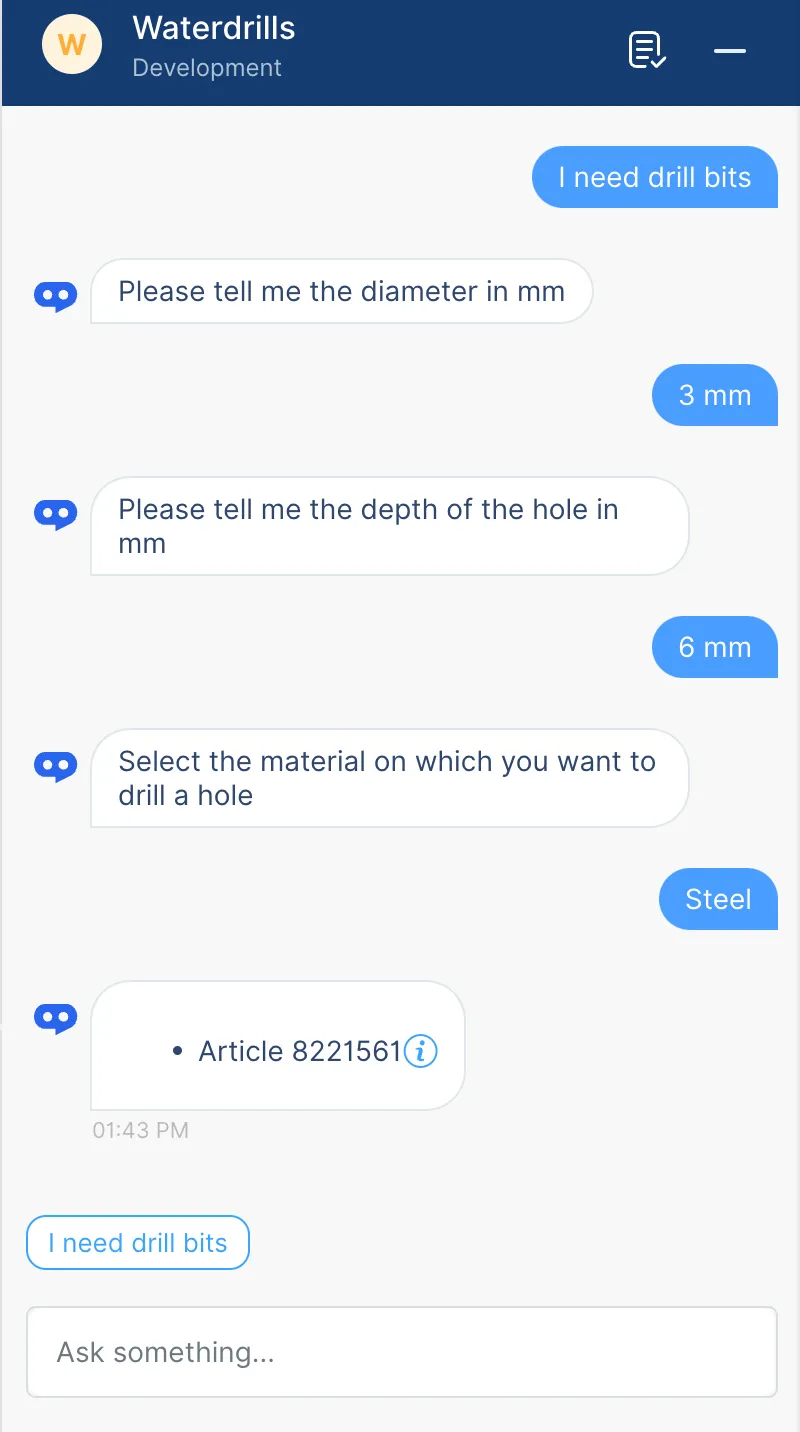
When you click on the info icon, the configured image, name and URL would be displayed.
- fields : The fields object lists the additional properties about the options. The fields object must contain the following attributes in the below format. This is mandatory only if the preview is configured.
copy[ { "label":"<the text to be displayed as the field name>", "value":"<the text to be displayed as the field value>" } ]
The key elements of the preview key are, header and fields. If the fields object is configured along with preview key, then the appropriate previews will be displayed when the user hovers on the respective options and there will be no info icon displayed in this case.
- buttons : Buttons are used to configure the operations to be performed on the options defined in the single and multiple selection list params. The buttons key should contain the following attributes in the below format.
copy[ { "id":"the unique id for the button", "label":"the text to be displayed as the button's label", "theme":"<positive | neutral | negative>" } ]
confirm
This contains a Map that specifies the confirmation message for action execution that should be prompted to the user. It is mandatory only if todo is set as confirm. The map value can contain only the below listed key :
- message : The confirmation message that should be prompted to the user.
assign
The value of the assign key is of type Map. This can be used to change the values of all the static params and the system defined arguments in runtime.
- clientData : Used to update the client data values based on the session data in runtime.
Implementation
Once you create an action for your ConvoKraft bot and choose to configure the response based on a business logic, you must define the sample sentences and respective params for it. Please make sure to follow the below listed steps to implement the Context handler function :
- After you configure and save the required sample sentences and params for an action, the Action’s details page will be displayed. Switch to Functions tab and enable the Context Handler function.
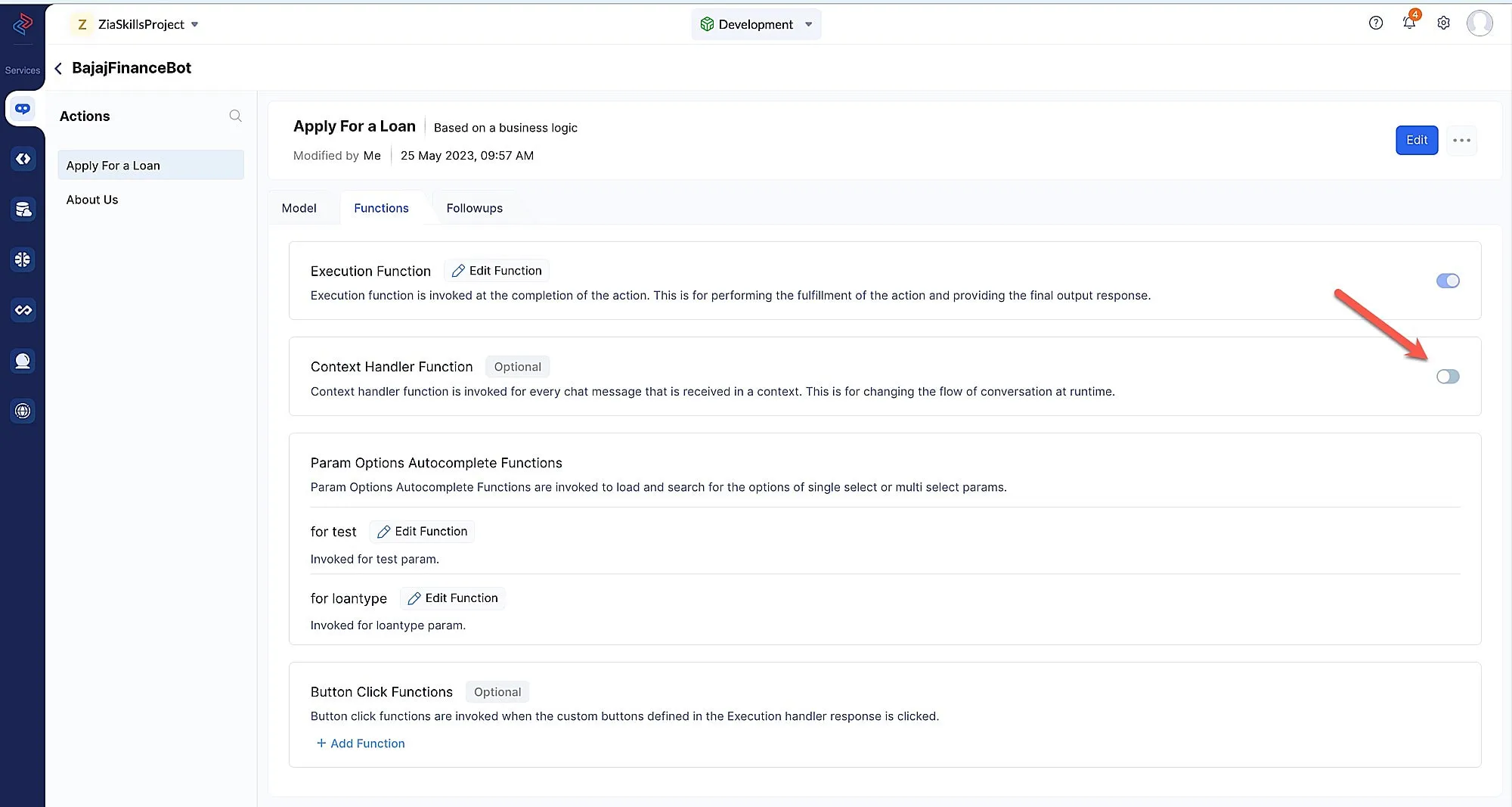
- You will be prompted with below message, Click OK to proceed.
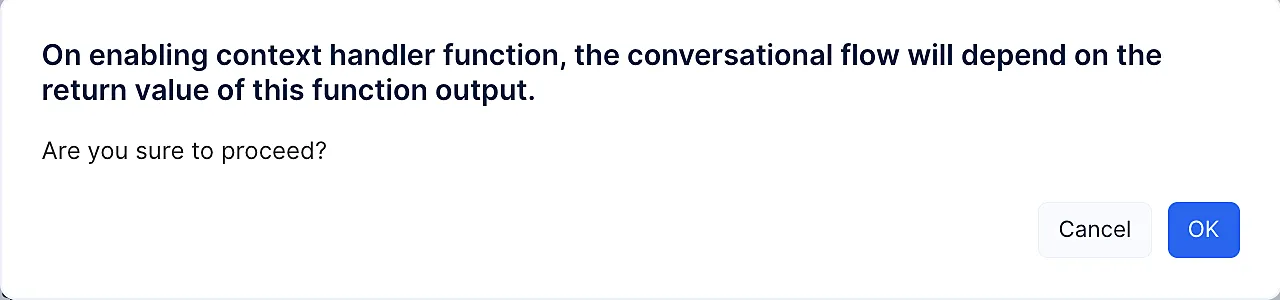
- The ConvoKraft Deluge editor will be opened where you can code your business logic and save the script. Learn more about the Deluge editor from this page. You can test the changes parallely using Test this bot feature.
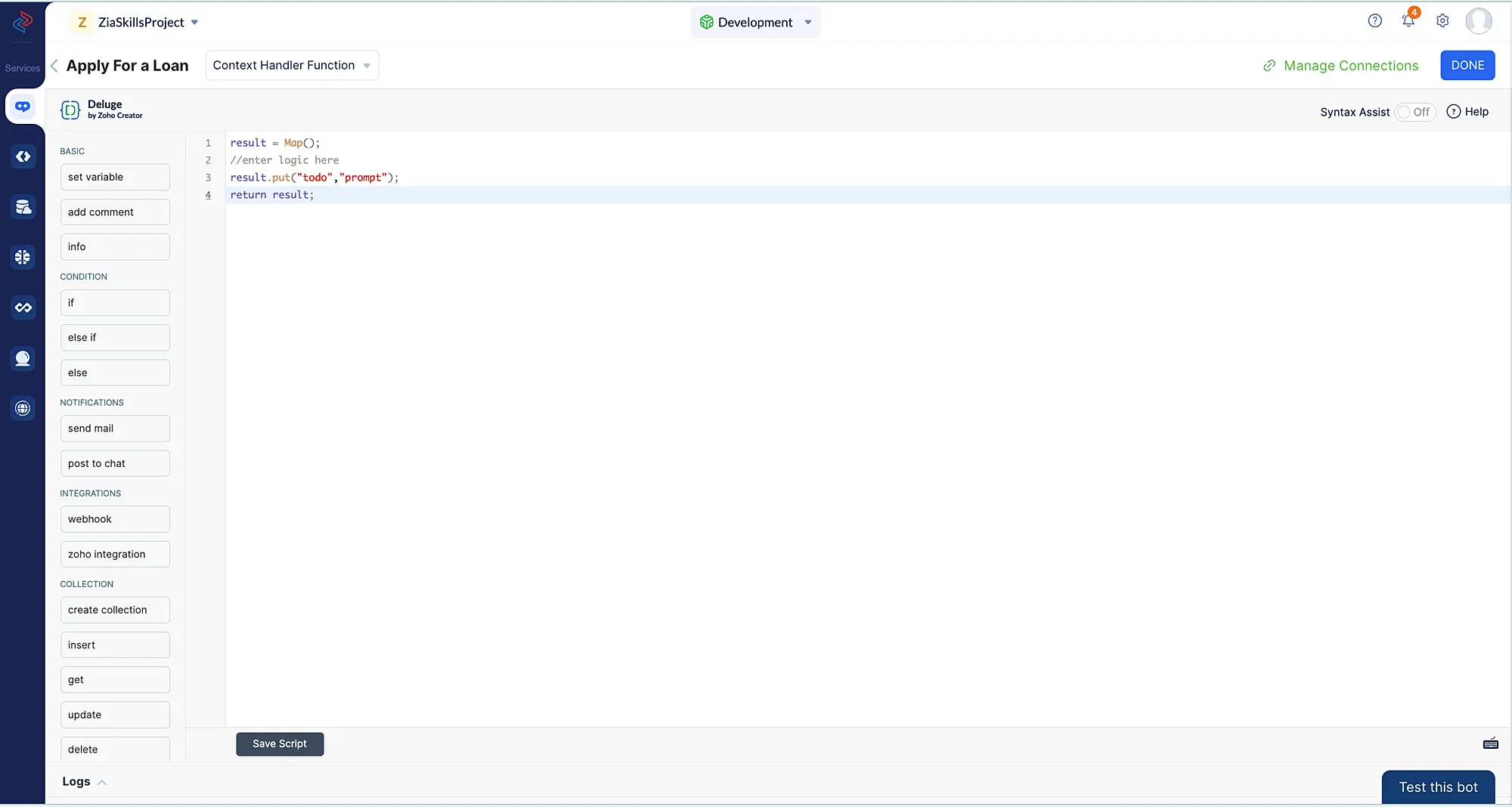
Last Updated 2025-02-19 15:51:40 +0530 +0530
Yes
No
Send your feedback to us