Configure the Event Function
We will now begin coding the WorkDrive Sync app by configuring the Event function.
The function directory functions/workdrivesync contains:
- The index.js main function file
- The catalyst-config.json configuration file
- Node modules
- package.json and package-lock.json dependency files
You can use any IDE to configure the function.
Install Packages for Node.js
The Node.js Event function requires three packages to be installed: axios, form-data, and fs.
axios
axios is a promise-based HTTP client that we will use to send asynchronous HTTP requests to the WorkDrive API endpoint to post files.
To install axios, navigate to the Node function’s directory (functions/workdrivesync) and execute the following command:
This will install the module.
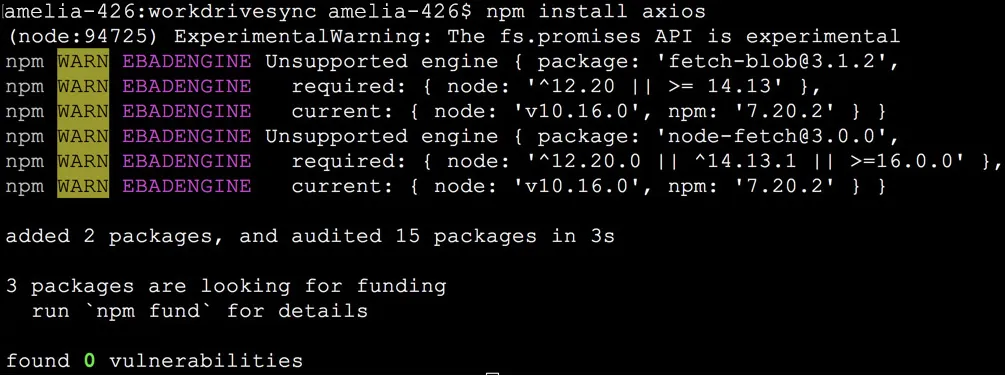
form-data
We will use form-data to upload the file to WorkDrive, after reading it from the event data sent in by the event listener.
To install form-data, navigate to the Node function’s directory (functions/workdrivesync) and execute the following command:
This will install the module.
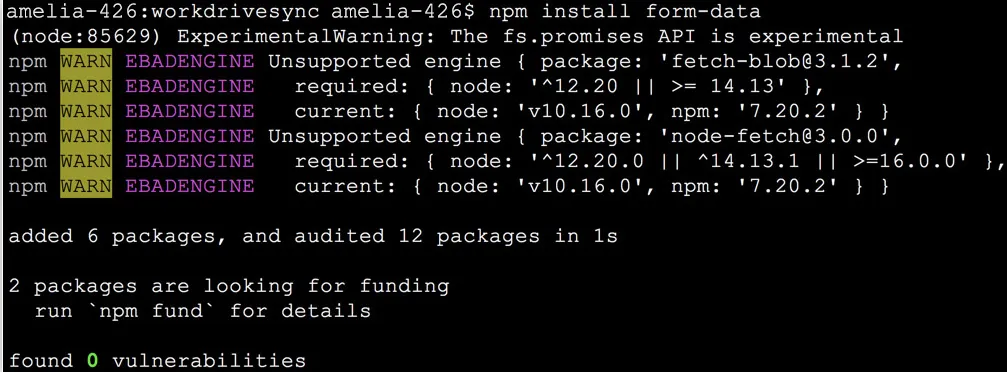
fs
The fs module enables us to access the physical file system and create a read stream to fetch the file from the event data.
To install fs, navigate to the Node function’s directory (functions/workdrivesync) and execute the following command:
This will install the module.
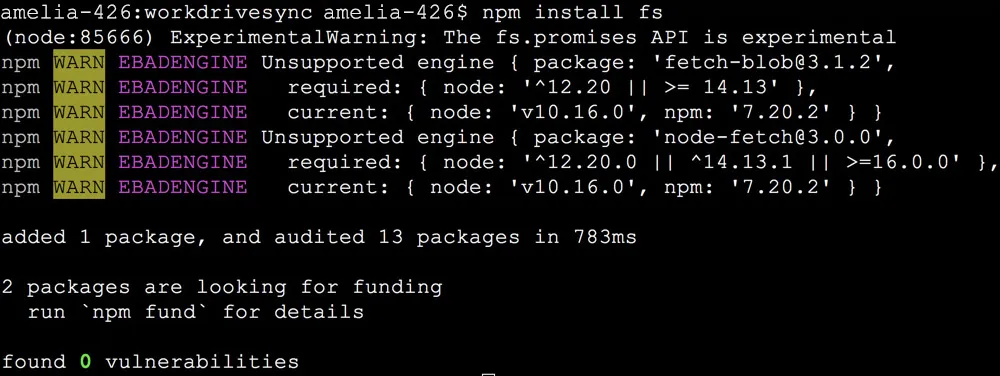
Information about these packages will also be updated in the package.json file of the Event function.
copy{ "name": "workdrivesync", "version": "1.0.0", "main": "index.js", "author": "emma@zylker.com", "dependencies": { "axios": "^0.21.4", "form-data": "^4.0.0", "fs": "^0.0.1-security", "zcatalyst-sdk-node": "latest" } }
You can now add the code in the function file.
Copy the code below and paste it in index.js located in functions/workdrivesync directory and save the file.
index.jscopyconst catalyst = require('zcatalyst-sdk-node'); const axios = require('axios').default; const FormData = require('form-data'); const fs = require('fs'); const credentials = { WorkDriveConnector: { client_id: '{{YOUR_CLIENT_ID}}', //Enter your Client ID client_secret: '{{YOUR_CLIENT_SECRET}}', //Enter your Client Secret auth_url: 'https://accounts.zoho.com/oauth/v2/token', refresh_url: 'https://accounts.zoho.com/oauth/v2/token', refresh_token: '{{YOUR_REFRESH_TOKEN}}' //Enter your Refresh Token } } const FOLDERID = '5m0kq28f2efdbc2464a37866cec7d6580cb47'; //Enter your WorkDrive Folder ID module.exports = async (event, context) => { try { const eventData = event.getRawData().events[0]; const eventType = eventData.event_config.api_name; const fileName = eventData.data.object_details[0].key; const app = catalyst.initialize(context); const query = `SELECT ROWID, WorkDriveFileID FROM FileVault where FileName='${fileName}'`; const queryResult = await app.zcql().executeZCQLQuery(query); console.log(queryResult); const ROWID = queryResult[0].FileVault.ROWID; const accessToken = await app.connection(credentials).getConnector('WorkDriveConnector').getAccessToken(); if (eventType === "stratus_object_uploaded") { const stratus = app.stratus(); const bucket = stratus.bucket("file-vault-demo"); // Replace your bucket name let fileStream = await bucket.getObject(fileName); const chunks = []; for await (const chunk of fileStream) { chunks.push(chunk); } const buffer = Buffer.concat(chunks); fs.writeFileSync(__dirname + '/' + fileName, buffer); var data = new FormData(); data.append('content', fs.createReadStream(__dirname + '/' + fileName)); const config = { method: 'POST', url: `https://workdrive.zoho.com/api/v1/upload?filename=${fileName}&override-name-exist=true&parent_id=${FOLDERID}`, headers: { 'Authorization': `Zoho-oauthtoken ${accessToken}`, ...data.getHeaders() }, data: data }; const response = await axios(config); console.log(response); const WorkDriveSync = 'Uploaded'; const body = response.data; const WorkDriveFileID = body.data[0].attributes.resource_id; const catalystTable = app.datastore().table('FileVault'); await catalystTable.updateRow({ WorkDriveFileID, WorkDriveSync, ROWID }); } else if (eventType === "stratus_object_deleted") { const WorkDriveFileID = queryResult[0].FileVault.WorkDriveFileID; const config = { method: "PATCH", url: `https://workdrive.zoho.com/api/v1/files/${WorkDriveFileID}`, headers: { Authorization: `Zoho-oauthtoken ${accessToken}`, Accept: "application/vnd.api+json", }, data: JSON.stringify({ data: { attributes: { status: "51", }, type: "files", }, }), }; console.log(config); await axios(config); const table = app.datastore().table("FileVault"); await table.deleteRow(ROWID); } context.closeWithSuccess(); } catch (err) { console.log(err); context.closeWithFailure(); } };
Note: After you copy and paste this code in your function file, ensure that you provide the following values in it as indicated by the comments:
- WorkDrive Folder ID in line 15: You can obtain this value by opening the folder you created in WorkDrive earlier. The URL contains the Folder ID of the WorkDrive folder. Copy the ID displayed after folders/ from the URL.
* Client ID in line 8 * Client Secret in line 9 * Refresh Token in line 12
The Event function is now configured.
Last Updated 2025-06-17 17:46:43 +0530 +0530