Configure the Cron Function
Next, we’ll begin coding the news application by configuring the cron function component.
The Cron function is initialized in Java, its directory, functions/NewsFetch, contains:
- The FetchNews.java main function file
- The catalyst-config.json configuration file
- Java library files in the lib folder
- .classpath and .project dependency files
We will be adding code in the FetchNews.java file.
You can use any IDE to configure the function.
As mentioned in the introduction, the cron function performs two tasks: making the API calls to the NewsAPI to fetch news, populating the news in Catalyst Data Store. The API calls are made using an API key provided by NewsAPI.
Register with NewsAPI
Before you code the cron function, you must register for a free developer subscription with NewsAPI and obtain the API key in the following way:
-
Visit https://newsapi.org/register.
-
Provide the required details and click Submit.
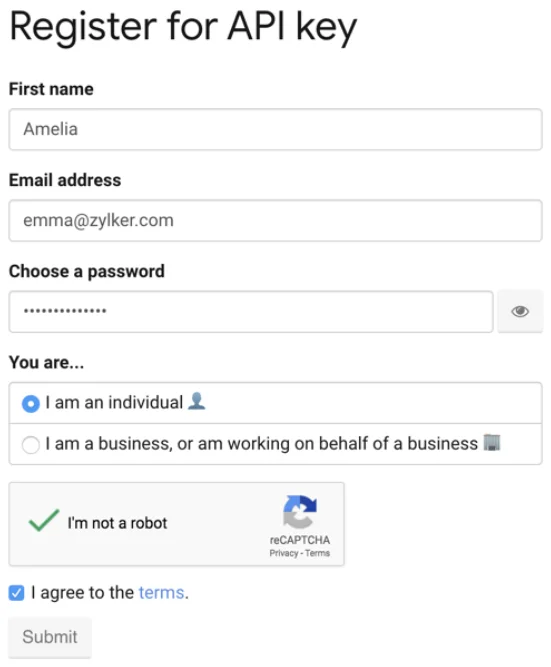
After your registration is complete, NewsAPI will provide you with an API key. You must use this in your cron function, as instructed after the code section.
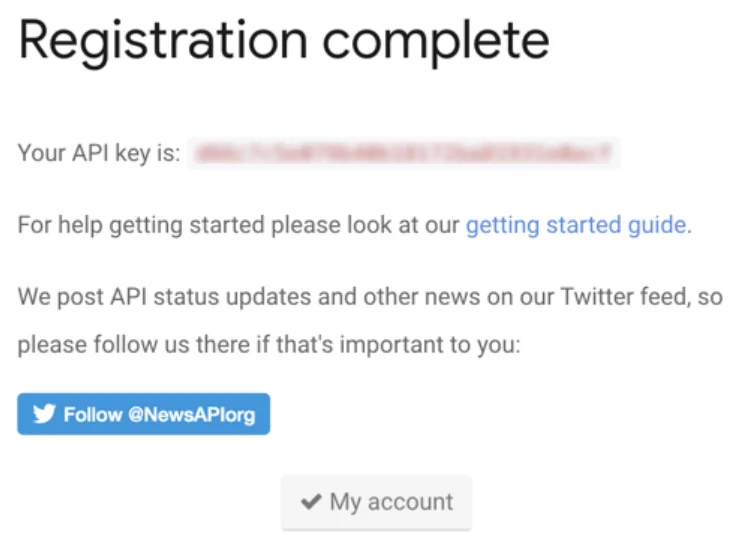
Add Function Code
You can copy the code below and paste it in FetchNews.java located in the functions/NewsFetch directory of your project and save the file.
FetchNews.javacopyimport java.util.ArrayList; import java.util.List; import java.util.logging.Level; import java.util.logging.Logger; import com.catalyst.Context; import com.catalyst.cron.CRON_STATUS; import com.catalyst.cron.CronRequest; import com.catalyst.cron.CatalystCronHandler; import com.zc.common.ZCProject; import com.zc.component.object.ZCObject; import com.zc.component.object.ZCRowObject; import com.zc.component.object.ZCTable; import com.zc.component.zcql.ZCQL; import org.json.JSONArray; import org.json.JSONObject; import okhttp3.HttpUrl; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; public class FetchNews implements CatalystCronHandler { private static final Logger LOGGER = Logger.getLogger(FetchNews.class.getName()); static String[] TABLENAME = { "HEADLINES", "BUSINESS", "ENTERTAINMENT", "HEALTH", "SCIENCE", "SPORTS", "TECHNOLOGY" }; // The names of the tables in the Data Store where the news items need to be stored static String COUNTRY = "US"; // Fetches the news items from the United States of America static String APIKEY = "
"; // Provide the API key you obtained from NewsAPI inside the quotations @Override public CRON_STATUS handleCronExecute(CronRequest request, Context arg1) throws Exception { try { ZCProject.initProject(); OkHttpClient client = new OkHttpClient(); // Fetches the breaking news of different categories with their headlines for (int i = 0; i < TABLENAME.length; i++) { // Builds the URL required to make the API call HttpUrl.Builder urlBuilder = HttpUrl.parse("http://newsapi.org/v2/top-headlines").newBuilder(); urlBuilder.addQueryParameter("country", COUNTRY); urlBuilder.addQueryParameter("apiKey", APIKEY); if (!TABLENAME[i].equals("HEADLINES")) { urlBuilder.addQueryParameter("category", TABLENAME[i]); } String url = urlBuilder.build().toString(); Request requests = new Request.Builder().url(url).build(); // Makes an API call to the News API to fetch the data Response response = client.newCall(requests).execute(); // If the response is 200, the data is moved to the Data Store. If not, the error is logged. if (response.code() == 200) { JSONObject responseObject = new JSONObject(response.body().string()); JSONArray responseArray = (JSONArray) responseObject.getJSONArray("articles"); // This method inserts/updates the news in the Data Store pushNewstoDatastore(responseArray, i); } else { LOGGER.log(Level.SEVERE, "Error fetching data from News API"); } //The actions are logged. You can check the logs from Catalyst Logs. LOGGER.log(Level.SEVERE, " News Updated"); } } catch (Exception e) { LOGGER.log(Level.SEVERE, "Exception in Cron Function", e); return CRON_STATUS.FAILURE; } return CRON_STATUS.SUCCESS; } private void pushNewstoDatastore(JSONArray responseArray, int length) throws Exception { String action = null; //Defines the ZCQL query that will be used to find out the number of rows in a table String query = "select ROWID from " + TABLENAME[length]; ArrayList rowList = ZCQL.getInstance().executeQuery(query); ZCRowObject row = ZCRowObject.getInstance(); List rows = new ArrayList<>(); ZCObject object = ZCObject.getInstance(); ZCTable table = object.getTable(TABLENAME[length]); // Inserts the data obtained from the API call into a list for (int i = 0; i < 15; i++) { JSONObject response = responseArray.getJSONObject(i); Object title = response.get("title"); String t = title.toString(); //Replace the " ' " string value to empty so that it doesnt affect the ZCQL Query t = t.replaceAll("'",""); Object url = response.get("url"); //Change the url Object to string for ZCQL update execution String u = url.toString(); row.set("title", title); row.set("url", url); // Obtains the number of rows in the table if (rowList.size() > 0) { Object RowID = rowList.get(i).get(TABLENAME[length], "ROWID"); String rowid = RowID.toString(); Long ID = Long.parseLong(rowid); //If there are no rows, the data is inserted, else the existing rows are updated row.set("ROWID", ID); rows.add(row); action = "Update"; String upd = "Update "+TABLENAME[length]+" set title ='"+t+"',url ='"+u+"' where ROWID = "+rowid; ZCQL.getInstance().executeQuery(upd); } else { action = "Insert"; table.insertRow(row); } } //The actions are logged. You can check the logs from Catalyst Logs. if (action.equals("Update")) { LOGGER.log(Level.SEVERE, TABLENAME[length] + " Table updated with current News"); } else if (action.equals("Insert")) { LOGGER.log(Level.SEVERE, TABLENAME[length] + " Table inserted with current News"); } } } Note: After you copy and paste this code in your function file, ensure that you replace value of APIKEY in the line 31 with the API key you obtained from NewsAPI.The Cron function is now configured. We will discuss the application’s architecture after you configure the client.
Last Updated 2025-05-26 17:57:12 +0530 +0530