Define Bot Responses
This section provides detailed information on the various response structures that can be configured in the ConvoKraft bot. You can configure responses for actions to provide direct answers or based on defined business logic. We will discuss the types of responses and their syntax in this section.
Direct Answer Response
When you create an action that responds based on the configured direct answer, you can format the answer based on your specific needs using the options listed below.
- Bold
- Italics
- Underline
- Strikethrough
- Highlight
- Heading
- Bullet list
- Numbered List
- Image
- Blockquote
- Table
You can use the formatting menus right above the editor, just click on them to apply the particular format. The screenshot below depicts an answer formatted with all the applicable styles.
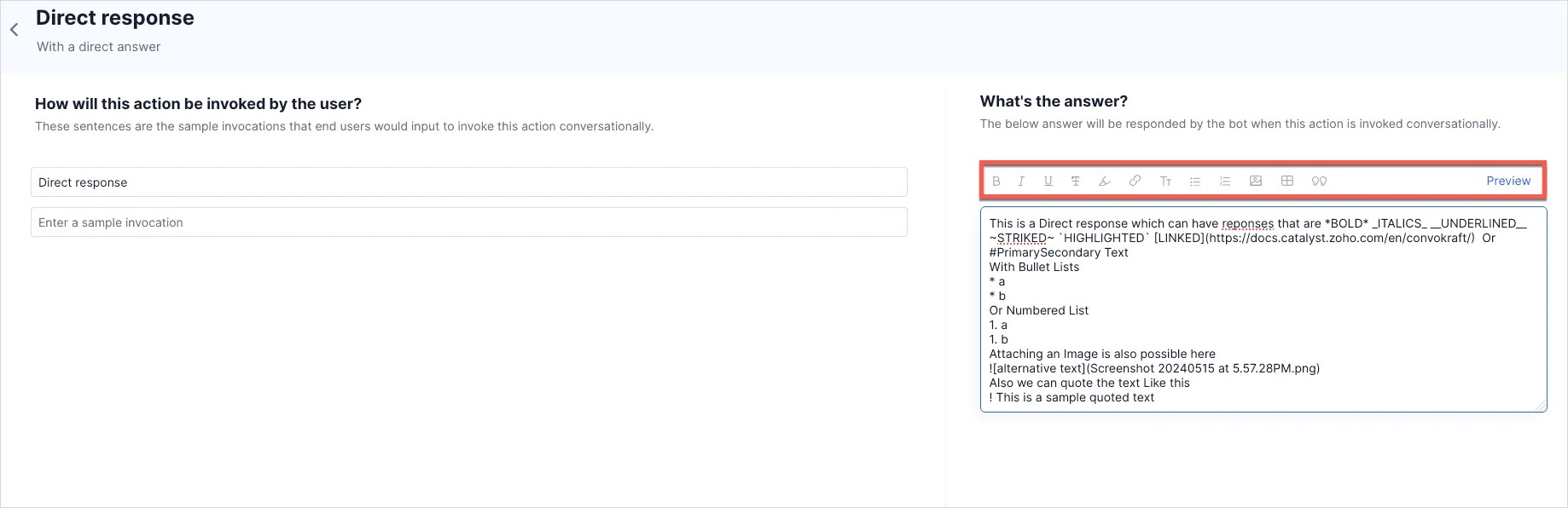
Click on Preview to view the output text of the formatted response.
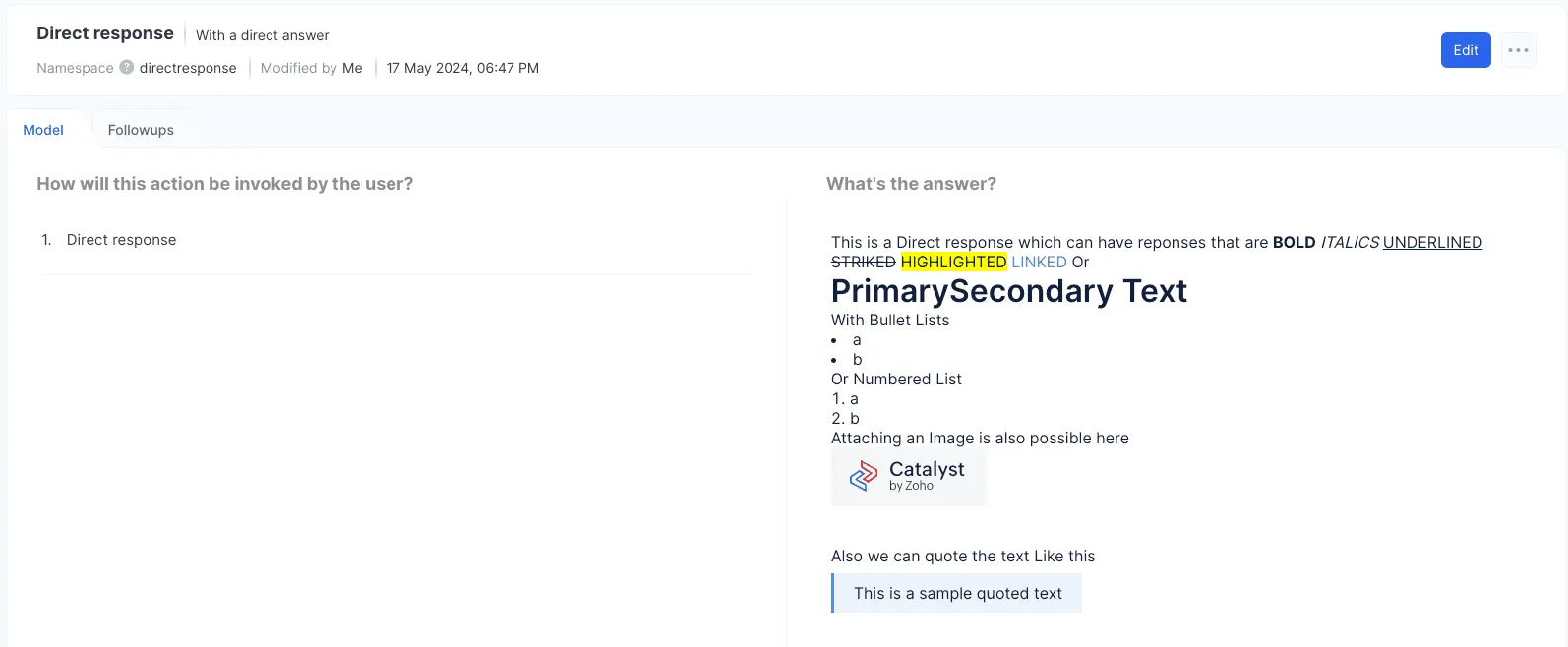
Respond based on a business logic
When creating an action that responds based on configured business logic, you can set up various responses in the Catalyst Integration Function, Deluge, or Webhooks using the syntaxes below. Let’s explore the different types of responses and their structures in detail.
Plain Text
To send a simple text message as a response to a user query in the ConvoKraft bot, please use the below structure.
JSON
copy{ "message": "This is a sample message response" }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("message", "This is a sample message response"); } return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.message="This is a sample message response"; return result; }
Python
copydef handle_execute_request(req_body): result = {} result['message'] = "This is a sample message response" return result
The response looks like this:
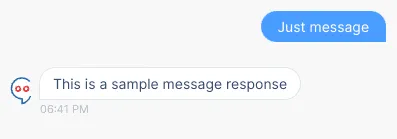
Card
To configure a card response in the ConvoKraft bot, please adhere to the following structures given below. You can learn more about configuring a card response specifically in the Deluge function, from this section.
Plain Card with Title and Note
This code snippet allows you to define a simple message in the card that includes a title and a note.
JSON
copy{ "message": "Plain Card response", "card": [ { "type": "title", "content": "This is a card with title" }, { "type": "note", "content": "This is a card with note" } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "title").put("content", "This is a card with title")) .put(new JSONObject().put("type", "note").put("content", "This is a card with note")));} return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"title", "content":"This is a card with title" }) result.card.push({ "type":"note", "content":"This is a card with note" }) return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "title", "content": "This is a card with title"}, {"type": "note", "content": "This is a card with note"} ] return result
The response looks like this:
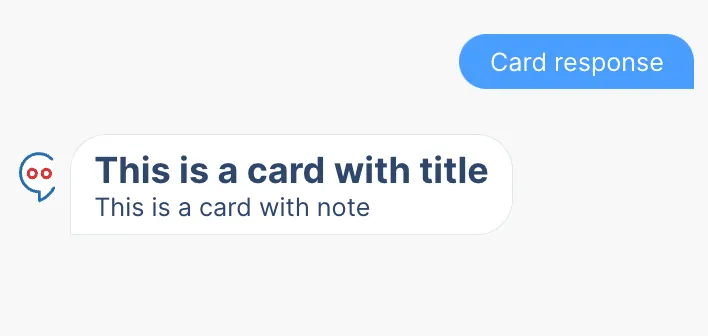
Bullet List Card
This code snippet allows you to define a bullet list within the card in the ConvoKraft response.
JSON
copy{ "message": "Bullet List RESPONSE", "card": [ { "type": "title", "content": "This is a title card for Bullet List" }, { "type": "list", "format": "bullet", "elements": [ { "label": "ABC" }, { "label": "DEF" } ] } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "title").put("content", "This is a title card for Bullet List")) .put(new JSONObject().put("type", "list").put("format", "bullet").put("elements", new JSONArray() .put(new JSONObject().put("label", "ABC")) .put(new JSONObject().put("label", "DEF")))));return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"title", "content":"This is a title card for Bullet List" }) result.card.push({ "type":"list", "format":"bullet", "elements":[ { "label":"ABC" }, { "label":"DEF" } ] }); return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "title", "content": "This is a title card for Bullet List"}, {"type": "list", "format": "bullet", "elements": [{"label": "ABC"}, {"label": "DEF"}]} ] return result
The response in the bot looks like this:
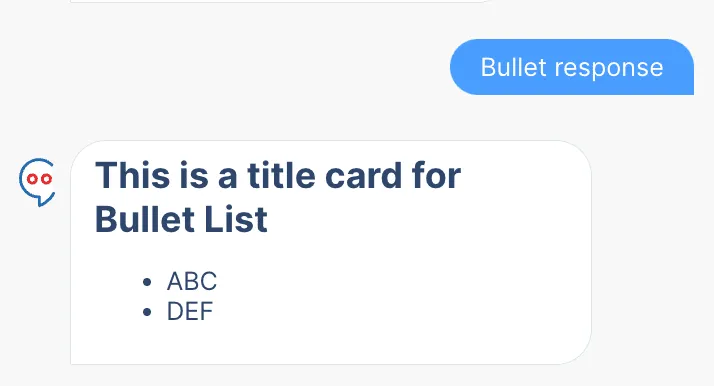
Numbered List Card
This code snippet allows you to define a numbered list within the card in the ConvoKraft response.
JSON
copy{ "message": "Numbered List RESPONSE", "card": [ { "type": "note", "content": "This is a note card with Numbered List" }, { "type": "list", "format": "numbered", "elements": [ { "label": "point no. 1" }, { "label": "point no. 2" } ] } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "note").put("content", "This is a note card with Numbered List")) .put(new JSONObject().put("type", "list").put("format", "numbered").put("elements", new JSONArray() .put(new JSONObject().put("label", "point no. 1")) .put(new JSONObject().put("label", "point no. 2"))))); return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"note", "content":"This is a note card with Numbered List" }) result.card.push({ "type":"list", "format":"numbered", "elements":[ { "label":"point no. 1" }, { "label":"point no. 2" } ] }); return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "note", "content": "This is a note card with Numbered List"}, {"type": "list", "format": "numbered", "elements": [{"label": "point no. 1"}, {"label": "point no. 2"}]} ] return result
The response in the bot looks like this:
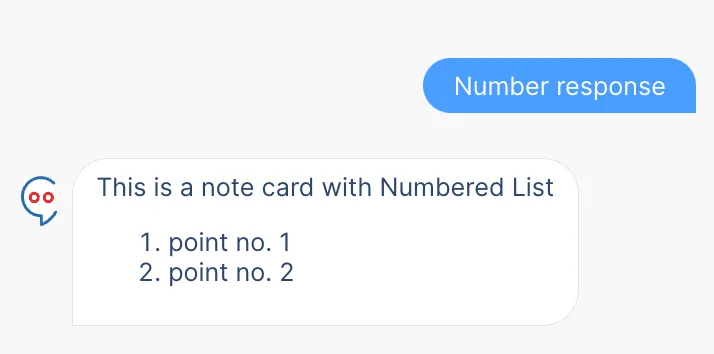
Link Response
This code snippet allows you to send a hyperlink within the card in the ConvoKraft response.
JSON
copy{ "message": "LINK RESPONSE", "card": [ { "type": "link", "text": "Click Here to Know about ZOHO", "content": "https://www.zoho.com" } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "link").put("text", "Click Here to Know about ZOHO").put("content", "https://www.zoho.com"))); return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"link", "text":"Click Here to Know about ZOHO", "content":"https://www.zoho.com" }) return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "link", "text": "Click Here to Know about ZOHO", "content": "https://www.zoho.com"} ] return result
The response in the bot looks like this:
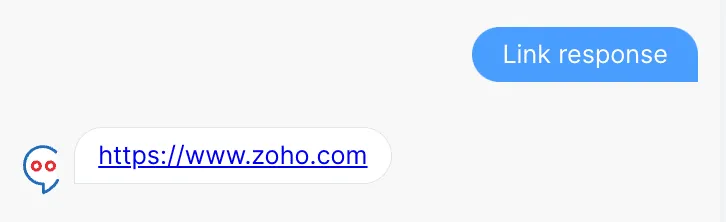
Table Response
This code snippet allows you to display a table with populated values within the card in the ConvoKraft response.
JSON
copy{ "message": "TABLE RESPONSE", "card": [ { "type": "table", "heading": "Convokraft Dummy Table", "columns": [ "Name", "AGE", "CITY" ], "rows": [ [ "BOB", "25", "SFO" ], [ "Evan", "28", "NY" ] ] } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "table").put("heading", "Convokraft Dummy Table").put("columns", new JSONArray() .put("Name").put("AGE").put("CITY")).put("rows", new JSONArray() .put(new JSONArray().put("BOB").put("25").put("SFO")) .put(new JSONArray().put("Evan").put("28").put("NY"))))); return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"table", "heading":"Convokraft Dummy Table ", "columns":[ "Name", "AGE", "CITY" ], "rows":[ [ "BOB", "25", "SFO" ], [ "Evan", "28", "NY" ] ] }) return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "table", "heading": "Convokraft Dummy Table", "columns": ["Name", "AGE", "CITY"], "rows": [["BOB", "25", "SFO"], ["Evan", "28", "NY"]]} ] return result
The response in the bot looks like this:
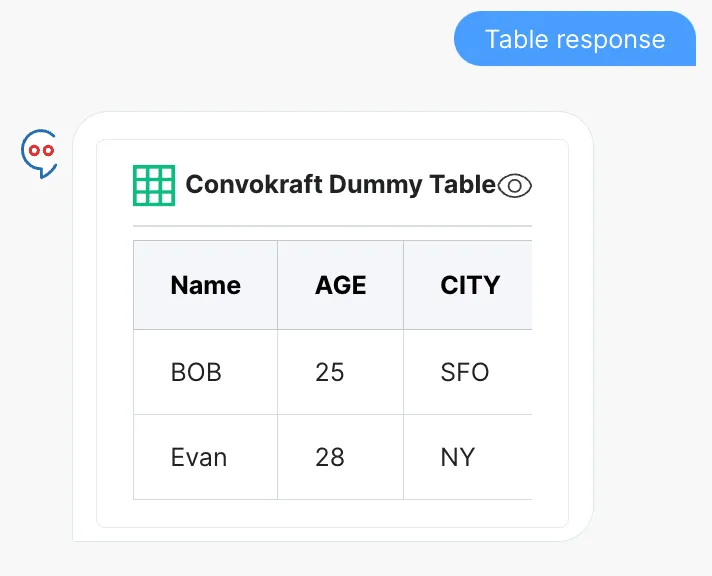
VCard Response
This code snippet allows you to define contact information including the first name, last name, company name and website within the card in the ConvoKraft response.
JSON
copy{ "message": "VCard Response", "card": [ { "type": "vcard", "info": { "image": "https://contacts.zoho.com/file?ID=53157432", "fields": [ { "First Name": "Prashaanth" }, { "Last Name": "Ramesh" }, { "Company": "Zoho" }, { "Website": "https://www.zoho.com" } ] } } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "vcard").put("info", new JSONObject().put("image", "https://contacts.zoho.com/file?ID=53157432").put("fields", new JSONArray() .put(new JSONObject().put("First Name", "Prashaanth")) .put(new JSONObject().put("Last Name", "Ramesh")) .put(new JSONObject().put("Company", "Zoho")) .put(new JSONObject().put("Website", "https://www.zoho.com")))))); return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"vcard", "info":{ "image":"https://contacts.zoho.com/file?ID=53157432", "fields":[ { "First Name":"Prashaanth" }, { "Last Name":"Ramesh" }, { "Company":"Zoho" }, { "Website":"https://www.zoho.com" } ] } }) return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "vcard", "info": {"image": "https://contacts.zoho.com/file?ID=53157432", "fields": [{"First Name": "Prashaanth"}, {"Last Name": "Ramesh"}, {"Company": "Zoho"}, {"Website": "https://www.zoho.com"}]}} ] return result
The response in the bot looks like this:
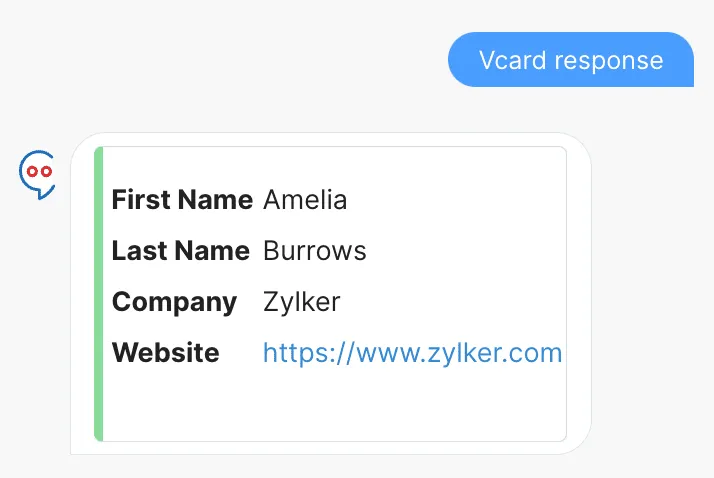
File Response
This code snippet allows you to send a file within the card in the ConvoKraft response.
JSON
copy{ "message": "FILE Response", "card": [ { "type": "file", "name": "SamplePDF", "format": "pdf", // File extension "content": "https://pdfobject.com/pdf/sample.pdf" } ] }
Java
copypublic class ExecuteHandler { public JSONObject handleExecuteRequest(JSONObject reqBody) { JSONObject jsonResponse = new JSONObject(); jsonResponse.put("card", new JSONArray() .put(new JSONObject().put("type", "file").put("name", "SamplePDF").put("format", "pdf").put("content", "https://pdfobject.com/pdf/sample.pdf")));return jsonResponse; }
Node.js
copyexport default function handleExecute(request) { let result={}; result.card=[]; result.card.push({ "type":"file", "name":"SamplePDF", "format":"pdf", // File extension "content":"https://pdfobject.com/pdf/sample.pdf" }) return result; }
Python
copydef handle_execute_request(req_body): result = {} result['card'] = [ {"type": "file", "name": "SamplePDF", "format": "pdf", "content": "https://pdfobject.com/pdf/sample.pdf"} ] return result
The response in the bot looks like this:
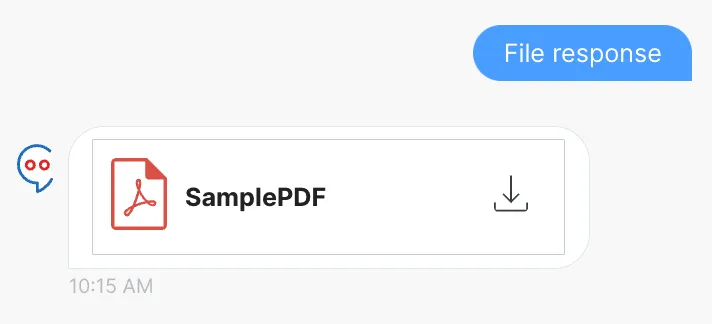
Last Updated 2025-02-19 15:51:40 +0530 +0530
Yes
No
Send your feedback to us