Configure the Advanced I/O Function
Next, we will begin coding the to-do list application by configuring the function component.
The function’s directory, functions/to_do_list_function contains:
- The index.js main function file
- The catalyst-config.json configuration file
- Node modules
- package.json and package-lock.json dependency files
You will be adding code in the index.js file.
The three APIs in the Advanced I/O function that handle the routing between the server and the Data Store are:
- GET /todo: To obtain the to-do list items from the TodoItems table in the Data Store
- POST /todo: To create and save a new list item in the Data Store
- DELETE /todo: To delete a list item from the Data Store
Install Express Framework for Node.js
We will need to use the Express framework to perform routing operations. To import the Express package in your function’s code, you must install the Express dependency in your system.
To install Express.js iin your local machine, navigate to the function’s home directory (functions/to_do_list_function) in your terminal and execute the following command:
This will install the Express module and save the dependencies.
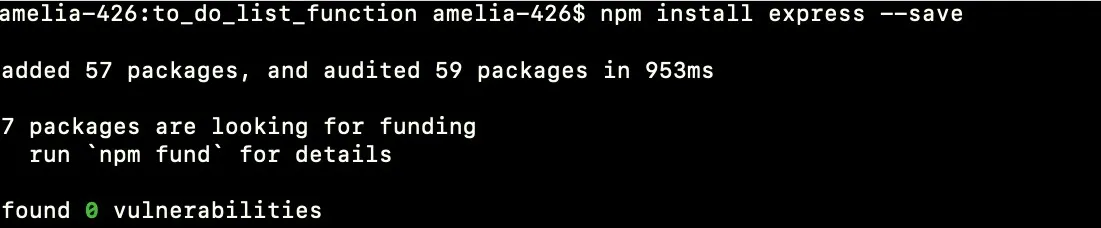
This information will also be updated in the package.json file.
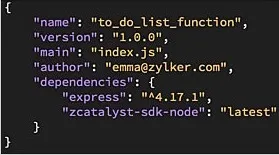
Next, let’s add the code in the function file. Copy the Node.js code and paste it in index.js in the functions/to_do_list_function directory of your project, and save the file. You can use any IDE of your choice to work with the application’s files.
index.jscopyconst express = require('express'); const catalystSDK = require('zcatalyst-sdk-node'); const app = express(); app.use(express.json()); app.use((req, res, next) => { const catalyst = catalystSDK.initialize(req); res.locals.catalyst = catalyst; next(); }); //GET API. Get existing tasks if any from the server. app.get('/all', async (req, res) => { try { const { catalyst } = res.locals; const page = parseInt(req.query.page); const perPage = parseInt(req.query.perPage); const zcql = catalyst.zcql(); const hasMore = await zcql .executeZCQLQuery(`SELECT COUNT(ROWID) FROM TodoItems`) .then((rows) => parseInt(rows[0].TodoItems.ROWID) > page * perPage); const todoItems = await zcql .executeZCQLQuery( `SELECT ROWID,Notes FROM TodoItems LIMIT ${ (page - 1) * perPage + 1 },${perPage}` ) .then((rows) => rows.map((row) => ({ id: row.TodoItems.ROWID, notes: row.TodoItems.Notes })) ); res.status(200).send({ status: 'success', data: { todoItems, hasMore } }); } catch (err) { console.log(err); res.status(500).send({ status: 'failure', message: "We're unable to process the request." }); } }); // POST API. Contains the logic to create a task app.post('/add', async (req, res) => { try { const { notes } = req.body; const { catalyst } = res.locals; const table = catalyst.datastore().table('TodoItems'); const { ROWID: id } = await table.insertRow({ Notes:notes }); res.status(200).send({ status: 'success', data: { todoItem: { id, notes } } }); } catch (err) { console.log(err); res.status(500).send({ status: 'failure', message: "We're unable to process the request." }); } }); // DELETE API. Contains the logic to delete a task. app.delete('/:ROWID', async (req, res) => { try { const { ROWID } = req.params; const { catalyst } = res.locals; const table = catalyst.datastore().table('TodoItems'); await table.deleteRow(ROWID); res.status(200).send({ status: 'success', data: { todoItem: { id: ROWID } } }); } catch (err) { console.log(err); res.status(500).send({ status: 'failure', message: "We're unable to process the request." }); } }); module.exports = app;
The functions directory is now configured.
Last Updated 2025-02-19 15:51:40 +0530 +0530