Configure the Advanced I/O Function
Let’s begin coding the Dialer app by configuring the function component.
The function’s directory,functions/dialer contains:
- The index.js main function file
- The catalyst-config.json configuration file
- Node modules
- package.json and package-lock.json dependency files
Install packages for Node.js
You will need to install two additional packages in the functions directory of your application before proceeding further.
Express
To import the Express Node.js package in the code, you must install the Express dependencies in your system. The express framework is used to manage routes and also respond to HTTP requests.
To install Express.js in your local machine, navigate to the function’s home directory (functions/dialer) in your terminal and execute the following command:
This will install the Express module and save the dependencies.
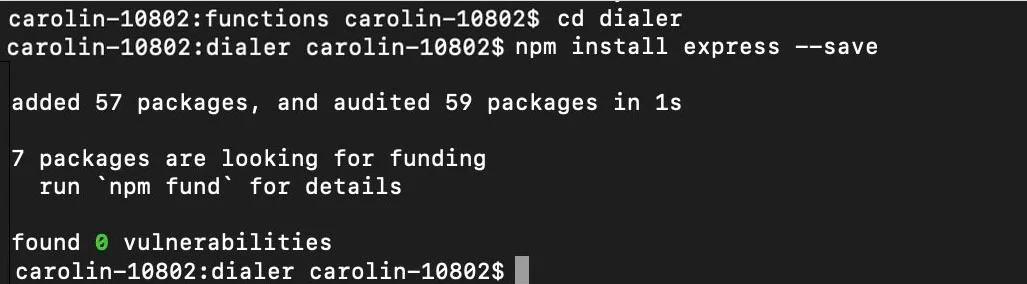
Twilio
You will also need to install the Twilio package for this tutorial. The Twilio library allows you to make HTTP requests to the Twilio API. Execute the below command to add the Twilio dependencies.
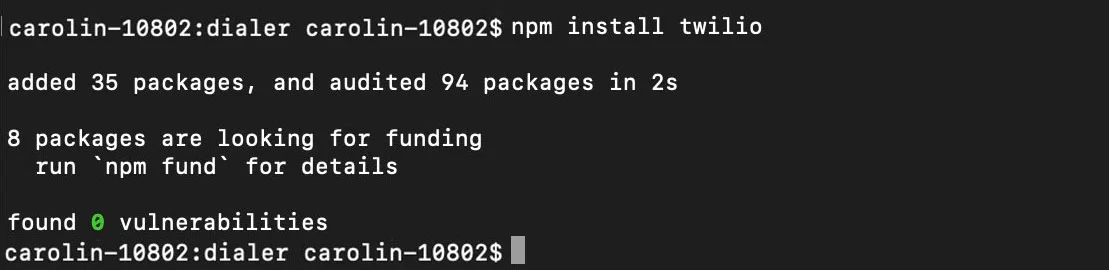
This information will also be updated in the package.json file.
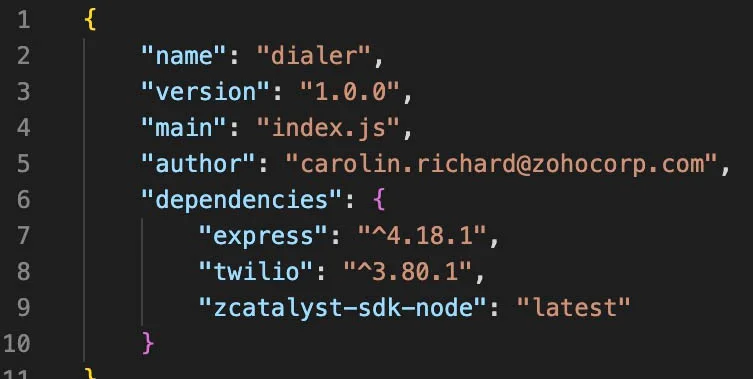
Next, let’s add the code in the function file. Copy the Node js code and paste it in index.js in the functions/dialer directory. You can use any IDE of your choice to work with the application’s files.
index.jscopyconst express = require("express"); const app = express(); var twilio = require('twilio'); const VoiceResponse = require("twilio").twiml.VoiceResponse; var catalyst = require('zcatalyst-sdk-node'); var YOUR_TWILIO_NUMBER = '+15612993019'; var accountSid = "xxxxxxxxxxxxxxx"; var authToken = "{{7xxxxxxxxxxxxxxxxx8}}"; var twilioClient = twilio(accountSid, authToken); var bodyParser = require('body-parser'); app.use(bodyParser.json()); // support json encoded bodies app.use(bodyParser.urlencoded({ extended: true })); // support encoded bodies app.post("/makeCall", async (req, res) => { try { var salesNumber = req.body.salesNumber; console.log(req.body) const catalystApp = catalyst.initialize(req); let rowData = { fromNumber: req.body.phoneNumber, toNumber: req.body.salesNumber }; console.log(rowData) const catalystTable = catalystApp.datastore().table('CallLog'); const data = await catalystTable.insertRow(rowData); var url = 'https://' + req.headers.host + '/server/dialer/outbound/' + encodeURIComponent(salesNumber); var options = { to: req.body.phoneNumber, from: YOUR_TWILIO_NUMBER, url: url, method: 'post', statusCallbackEvent: ['answered', 'completed'], statusCallback: 'https://' + req.headers.host + '/server/dialer/statusCheck?ROWID=' + data.ROWID, statusCallbackMethod: 'POST' }; twilioClient.calls.create(options).then((call) => { res.status(200).send({ message: 'Call Initiated' }); }).catch((error) => { console.log(error); res.status(500).send({ "error": error }); }); } catch (e) { console.log("Exception while making a Call ::" + e); res.status(500).send({ "error": 'Internal Server Error Occured. Please Try again after sometime.' }); } }); app.post('/outbound/:salesNumber', function (req, res) { try { var salesNumber = req.params.salesNumber; var twimlResponse = new VoiceResponse(); twimlResponse.say('Thanks for trying out the Catalyst Dialer App. Please hold on so that we can connect with your Customer... ', { voice: 'alice' }); twimlResponse.dial(salesNumber); res.status(200).send(twimlResponse.toString()); } catch (e) { console.log("Exception while making Outbound Call ::" + e); res.status(500).send({ "error": 'Internal Server Error Occured. Please Try again after sometime.' }); } }); app.post('/statusCheck', async (req, res) => { try { const catalystApp = catalyst.initialize(req); if (req.body.CallStatus === "completed") { let updatedRowData = { callDuration: req.body.CallDuration, callStatus: req.body.CallStatus, calledCountry: req.body.CalledCountry, calledState: req.body.CalledState, timeOfCall: req.body.Timestamp ROWID: req.body.ROWID }; console.log(updatedRowData) const catalystTable = catalystApp.datastore().table('CallLog'); await catalystTable.updateRow(updatedRowData); } res.status(200).send(' StatusCheck Completed'); } catch (e) { console.log("Exception while checking status :: " + e); res.status(500).send({ "error": 'Internal Server Error Occured. Please Try again after sometime.' }); } }); app.get('/getLogs', async function (req, res) { try { const catalystApp = catalyst.initialize(req); const query = 'SELECT callDuration,callStatus,calledCountry,timeOfCall,fromNumber,toNumber FROM CallLog'; const queryResult = await catalystApp.zcql().executeZCQLQuery(query); res.status(200).send(queryResult); } catch (e) { console.log("Exception while getting Call Logs ::" + e); res.status(500).send({ "error": 'Internal Server Error Occured. Please Try again after sometime.' }); } }); module.exports = app;
Note:Please make sure you add your Twilio accountID, associated Twilio phone number ,and auth token values in lines 6, 7, and 8.The functions directory is now configured. We will discuss the function and the client code in the next section.
Last Updated 2025-02-19 15:51:40 +0530 +0530